Custom Fields

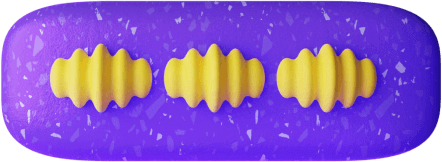


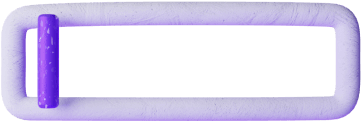
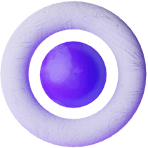
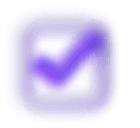
Allows custom fields in Formally forms, so that you can extend Formally to support any field type
Intro
Typically Form Builders only support the form field types that are built in, such as text boxes or checkboxes.
If a Form Builder doesn't support (e.g.) a 'map location picker' then there's typically no way to add that.
Formally can have placeholders for form fields (called 'Custom Fields') which –when embedded– can be resolved to arbitrary React components. It is up to the developer/integrator to implement the Custom Field resolver.
Custom Fields work with Repeaters, Conditionals, translations (for form text such as labels, hints, validation etc), and all other Formally features.
Requirements
- SDK licence;
- A developer who knows a bit of React;
- Only available when embedded via React for now, not plain JavaScript;
- Custom Fields are only supported when embedded on your site. They aren't supported when using Formally Hosted Forms (ie, https://MadeWithFormally.com ).
Usage in the Form Builder
When using the Form Builder you can create new custom fields on the 'Organisation Settings' page. These are shared across all forms in your organisation.
Take note of the 'Custom Field Id' as this is the identifier that developers will implement a Custom Field resolvers for.
Authoring Custom Field resolvers
The developer can then resolve these with the customFields
prop
of <Formally>
:
<Formally
formBuilderId="your-formbuilder-id"
customFields={{
myCustomFieldId: myComponent,
}}
/>;
const myComponent = ({
inputFieldId,
locale,
node,
name,
value,
fieldState,
label,
hint,
error,
onChange,
onBlur,
}) => {
// your custom component goes here and
// it can be anything you want.
//
// Use the 'value' prop, and 'onChange' to
// update the value in Formally. Use 'onBlur'
// to trigger validation checks. Use Formally
// features like Repeaters or Conditionals
//
// For accessibility reasons be sure to use
// the 'label' prop or render your own
// <label htmlFor={inputFieldId}>
return (
<>
{label}
<input
id={inputFieldId}
value={value}
onChange={(e) => onChange(e.target.value)}
onBlur={onBlur}
/>
</>
);
};
See also
React JSX <CustomField>.Using JSON?
When authoring JSON make an item NodeCustomField
in your form:
<CustomField>
's props
Prop | About | Type |
---|---|---|
component | a The | string |
hideInAnswerSummary | Whether to hide any answers below this. | boolean |
hintHtml | Localisable HTML: hint content E.g.
| LocalisedHtml |
isRequired | Whether users are required to fill in this form field. The custom | boolean |
labelHelpPopoutContentButtonLabel | Localisable text: Popout Content button label | LocalisedPlainText |
labelHelpPopoutContentModalContentHtml | Localisable text: Popout Content modal title | LocalisedHtml |
labelHelpPopoutContentModalHeadingHtml | Localisable text: Popout Content modal title | LocalisedHtml |
labelHtml | Localisable HTML: Label content E.g.
| LocalisedHtml |
name | The Name of the form control. Submitted with the form as part of a name:value pair in the | string |
validationErrorInvalidHtml | Localisable HTML: invalid validation content | LocalisedHtml |
validationErrorRequiredHtml | Localisable HTML: required validation content | LocalisedHtml |
id | Component Id. Must be unique within the form. | string |
meta | Arbitrary metadata on this node. This is useful when developing custom controls as it allows you to pass down metadata/extensions. | string |
hasChildrenById | Non-editable. Used to indicate that this component doesn't have children. | false |
hasTagsById | Non-editable. Used to indicate that this component isn't a form field with tags by id. | false |
isFormField | Non-editable. Used to indicate that this component is a form field. | true |
isMultichoice | Non-editable. Used to indicate that this component isn't a multichoice form field like | false |
type | Non-editable. Type of component of | "CustomField" |